6.2.1 - SUI Panels
How to create a panel
A panel (SPanel in SUI) is the main window in which others UI elements are placed into.
To create a new panel we need to use the RegisterNewPanel method provided by the SUI class.
If you don't see it add using SUI
and using static SUI.SUI
at the top of the cs file.
The methods takes two parameters (three in reality)
- The panel id: unique identifier of the panel, used to toggle it, refer to it etc. This must be unique since two mods sharing the same id could interfere each other
- A boolean value: used to specify if the panel should show the mouse cursor and receive input from it
static bool receiveInput;
public static void Create()
{
RegisterNewPanel("panel id", receiveInput);
}
If you execute the code above you will see that no panel will be displayed on screen. That's because it needs more informations to being displayed. Those are:
- Anchor type
- Color
- Size or Margin
Anchor defaults to AnchorType.Fill. If you just add a color to the panel you will see that it will expand to occupy all the screen (as the name says: fill). Apparently changing the size of panel has no effect if the panel AnchorType type is set to fill, so we can try to change it to AnchorType.MiddleCenter and then apply a color and a size to it so we get the 1280x720 blue panel below.
RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue).Size(1280, 720);
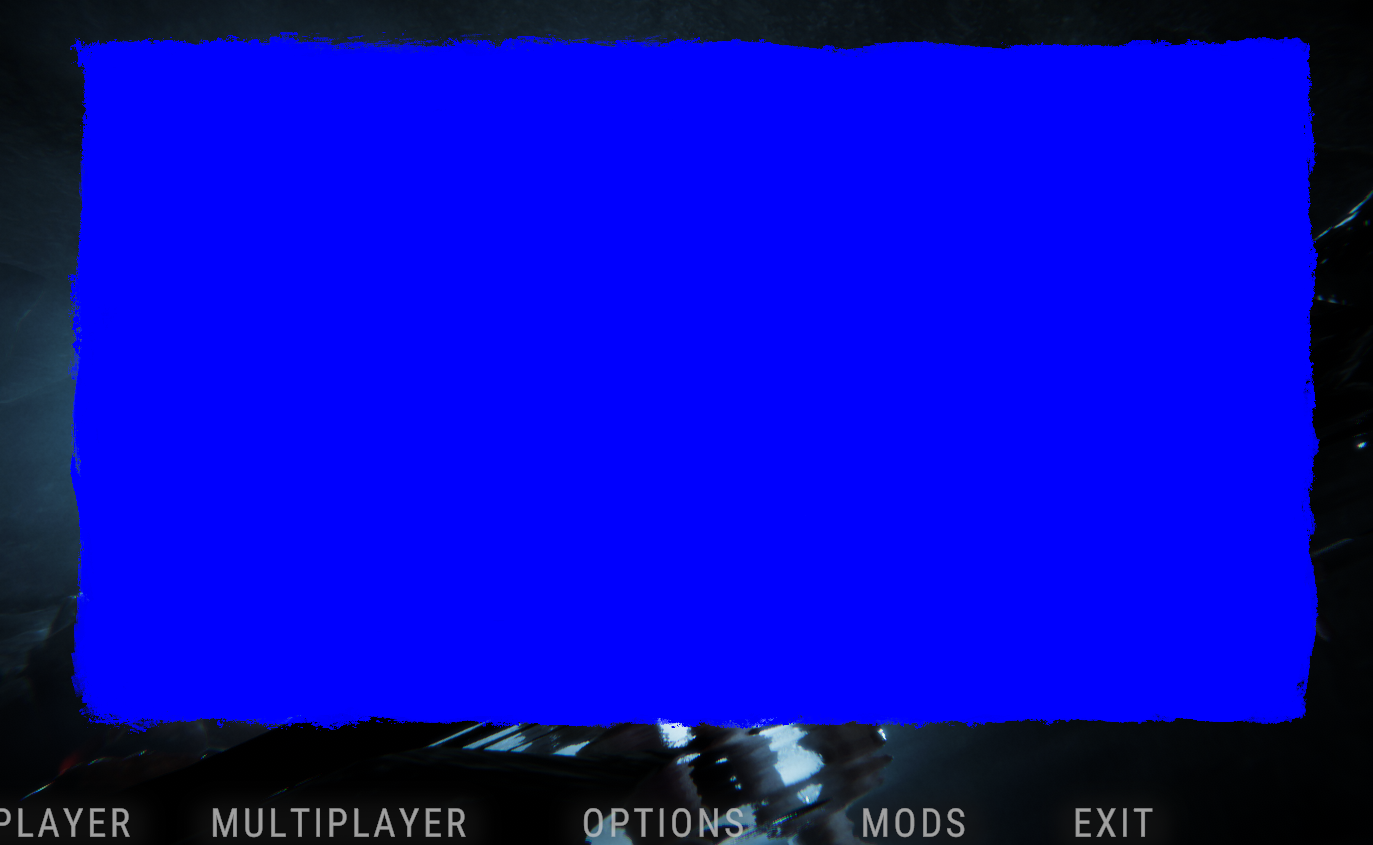
If we leave the AnchorType as default (AnchorType.Fill) you can also use the .Margin property to give a size to the panel starting from the screen borders. In the example below we have have a panel which starts 50px from the left, 50px from the right, 200px from the top and 200px from the bottom of the screen.
RegisterNewPanel("panel id", true).Background(Color.blue).Margin(50, 50, 200, 200); // AnchorType is implicitly set to fill when not specified
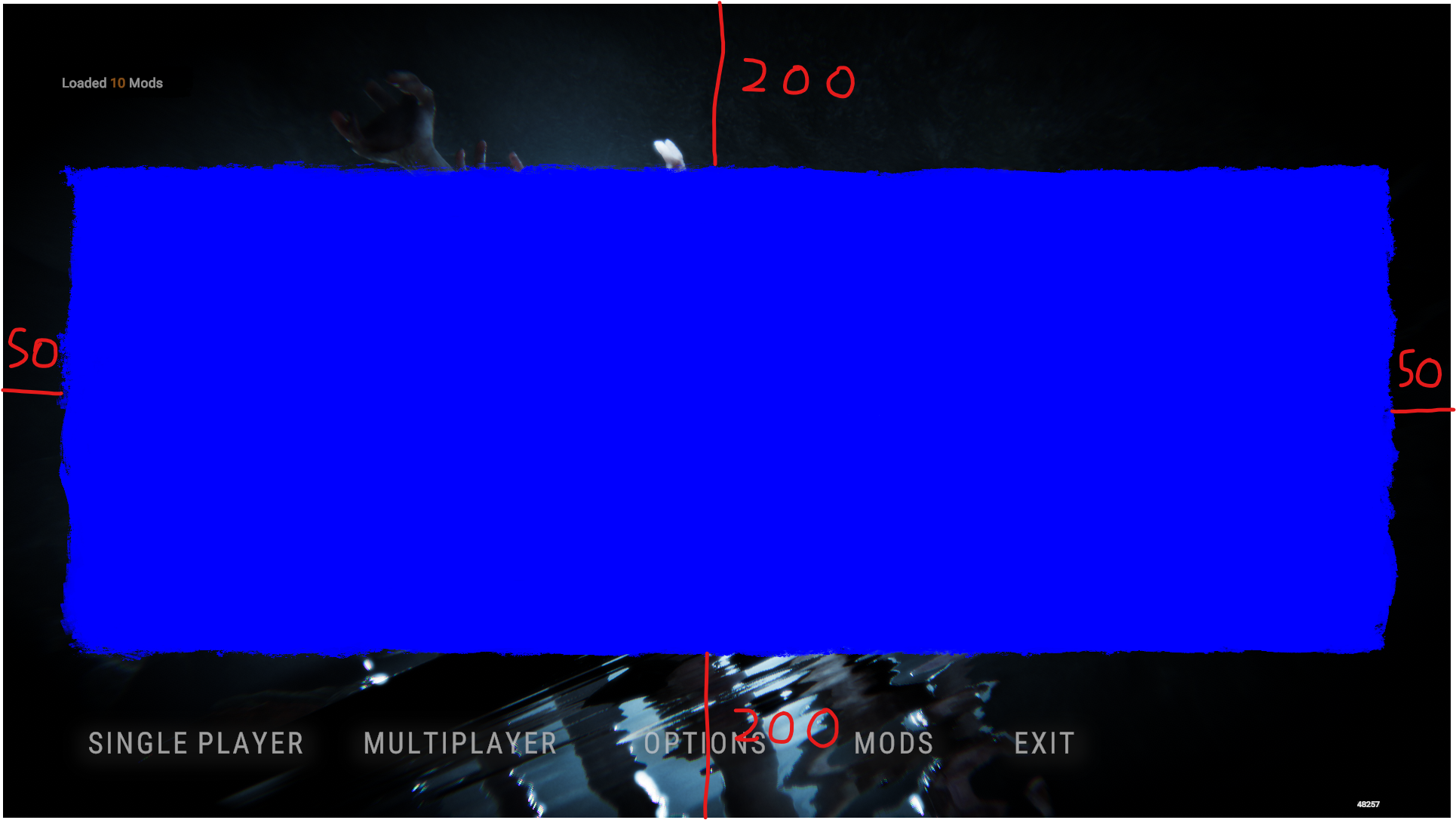
Placing the panel topleft, on the right, at the bottom etc.
To move the panel to another place we can always use the .Anchor property. We can for example move it top the top left corner of the screen like this:
RegisterNewPanel("panel id", true).Anchor(AnchorType.TopLeft).Background(Color.blue).Size(1280, 720);
But you will quickly see half of the panel is outside of the screen. To fix this I personally found two ways:
- Manually adjust the position by moving the panel half size of it to the right and to the bottom
- Change the pivot of the panel
// fixing panel position by adjusting it's position
RegisterNewPanel("panel id", true).Anchor(AnchorType.TopLeft).Background(Color.blue).Size(1280, 720).Position(640 /*moving right*/, -360 /*moving down*/);
// fixing panel position by changing it's pivot
RegisterNewPanel("panel id", true).Anchor(AnchorType.TopLeft).Background(Color.blue).Pivot(0, 1);
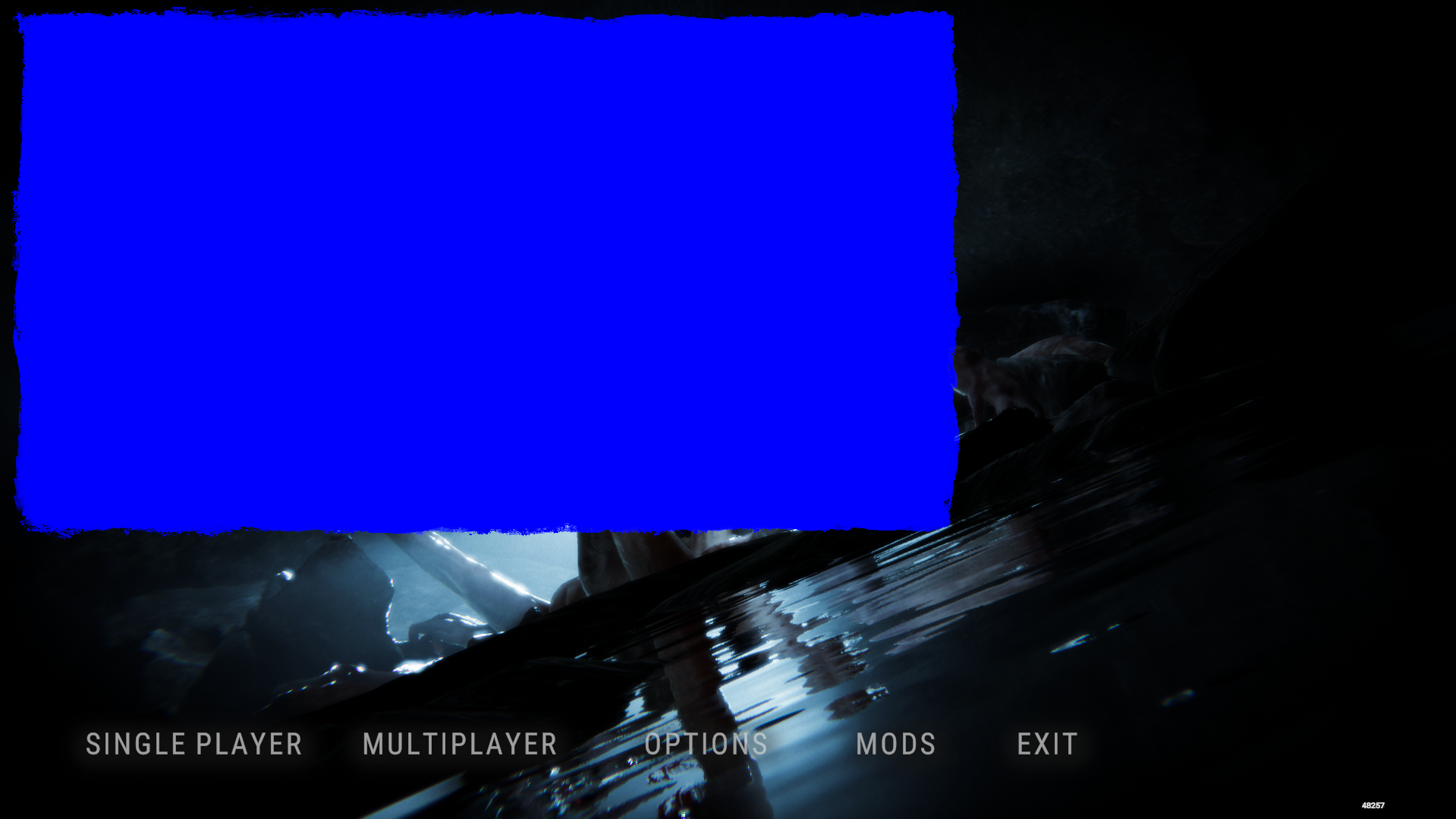
Why Pivot(0, 1)?
We can take a look at the screen below
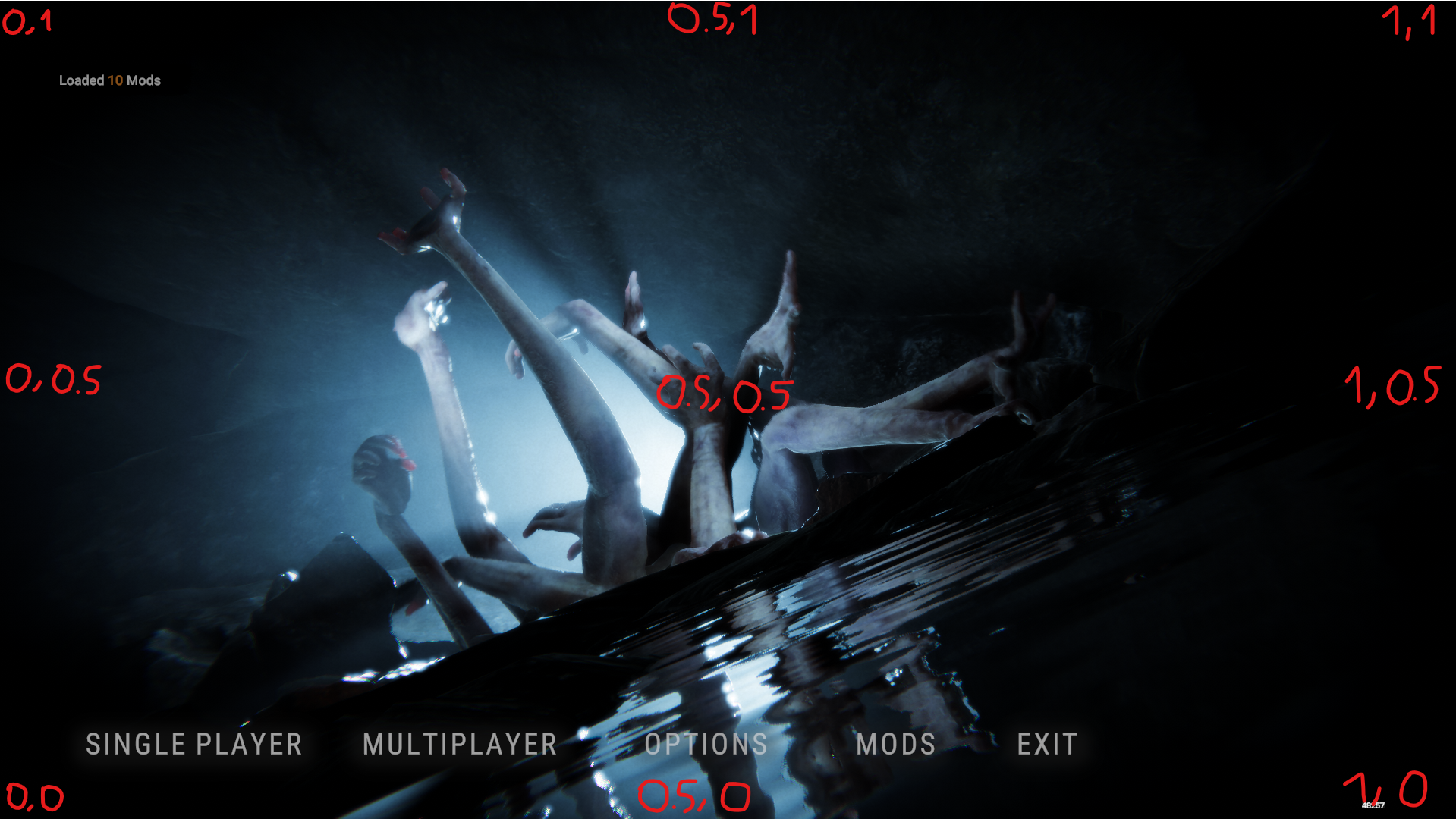
As you can see 0,1 corresponds to the top left corner and that's why we set a pivot of 0,1 (x: 0, y: 1).
Try to change the panel AnchorType to top right and the pivot value to 1,1 and you will see the panel is correctly placed to the top right of the screen.
I will leave the list of the pivot values for each AnchorType below:
AnchorType.TopLeft => (0, 1),
AnchorType.MiddleLeft => (0, 0.5f),
AnchorType.BottomLeft => (0, 0),
AnchorType.TopCenter => (0.5f, 1),
AnchorType.MiddleCenter => (0.5f, 0.5f),
AnchorType.BottomCenter => (0.5f, 0),
AnchorType.TopRight => (1, 1),
AnchorType.MiddleRight => (1, 0.5f),
AnchorType.BottomRight => (1, 0),
Showing / Hiding a panel
To show and hide a panel we have two ways:
- Using the TogglePanel method of the SUI class
- Using the .Active method on the panel itself
RegisterNewPanel("panel id", true).Anchor(AnchorType.TopLeft).Background(Color.blue).Pivot(0, 1);
TogglePanel("panel id"); // will hide the panel if visible or show it if hidden
TogglePanel("panel id", false); // will hide the panel
TogglePanel("panel id", true); // will show the panel
var panel = RegisterNewPanel("panel id", true).Anchor(AnchorType.TopLeft).Background(Color.blue).Pivot(0, 1);
panel.Active(false); // will hide the panel
panel.Active(true); // will show the panel
Styling the panels
To change the theme of the panel we use the .Background property, which is also used to change the panel color as seen before. There are many styles which are defined in the EBackground enumerator. The default one is EBackground.Sons, which is also the one used in the examples above. Others can be EBackground.None where the borders are straight or EBackground.RoundedStandard where they have some rounding:
// A panel with straight borders (EBackground.None)
RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.None).Size(1280, 720);
// A panel with rounded borders (EBackground.RoundedStandard)
RegisterNewPanel("panel id", true).Anchor(AnchorType.MiddleCenter).Background(Color.blue, EBackground.RoundedStandard).Size(1280, 720);